Finito!
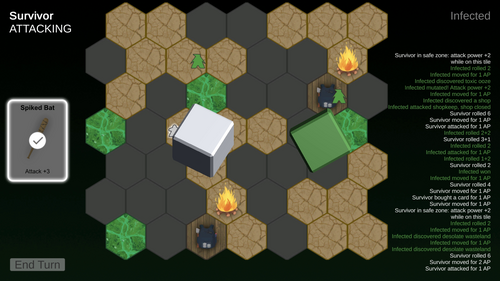
Well, that was fun! The enemy AI came together quite quickly. I know it probably seems a bit odd to leave that till the very end, but I knew it would be arguably the easiest part of the whole thing.
In the end the enemy logic looks like this:
- Always start by attacking on current tile, if mutated
- Then try to mutate (find nearby toxic ooze)
- Then move to attack with a mutated unit
- Then try to move to an undiscovered hex
- Finally, fall back to randomly moving to an empty hex tile
Sadly, towards the very end I noticed that my distance calculation was completely wrong... 🤦♂️ I was using Vector3Int.Distance which works fine for grids but obviously doesn't account for the even/odd nature of a hex grid. So I whipped together a quick solution based on this excellent blog post:
private Vector3Int OddrToCube(Vector3Int pos)
{
var x = pos.x - (pos.y - (pos.y & 1)) / 2;
var y = pos.y;
var z = -x - y;
return new Vector3Int(x, y, z);
} private int CubeDistance(Vector3Int a, Vector3Int b)
{
return (Mathf.Abs(a.x - b.x) + Mathf.Abs(a.y - b.y) + Mathf.Abs(a.z - b.z)) / 2;
} public int AdjustedDistance(Vector3Int a, Vector3Int b)
{
Vector3Int ca = OddrToCube(a);
Vector3Int cb = OddrToCube(b);
return CubeDistance(ca, cb);
}
I also took the opportunity to add some variety in the survivor cards by varying the attack point modifiers.
And with that, my 7DLR jam entry is complete! Sadly this will have to go on the shelf for a bit, but I look forward to possibly expanding on it in the future. Thanks for following!
Files
Infector
Tabletop inspired roguelike
More posts
- Two days remain...Mar 04, 2020
- The halfway mark!Mar 03, 2020
- Weekend progressMar 02, 2020
- 7DRL here we go!Mar 01, 2020
Leave a comment
Log in with itch.io to leave a comment.